How to alert me if files haven’t moved
Q: We haven’t spoken in a while which means file mover is working great. I have a question though, can file mover alert me if files haven’t moved from a folder it has delivered some files to? I’m having some different trouble with an application which has just started. One input folder is sometimes not moving the files into the next application, when you click on the folder in this application it looks empty, but when you look at the folder in the OS you can see its got loads of files in.
I would love to have an alert to say that there are 5 or more files in this folder, is there a problem.
A: This is possible using our scripting option. Please have a look at the following screenshots. If you need any help, please let us know.
- Important, the “Function” must be set to COPY
- We added a Windows folder as Source (but any other Source types should work also)
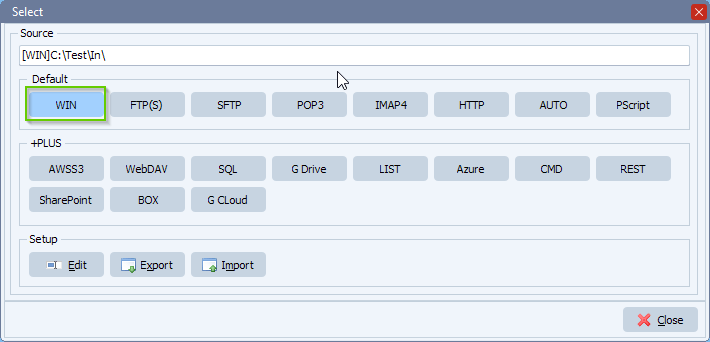
- In our example we want to count files older than 5 minutes so we added the following “File Date Include” filter:
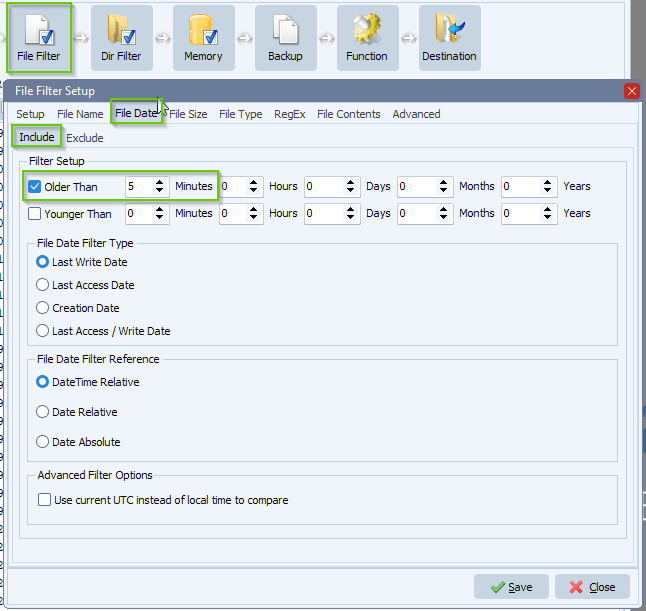
- As Destination we added our “Pascal Script” option. The script will use an internal Counter psVIA (pascal script Variable Integer A) to count the amount of files we found.
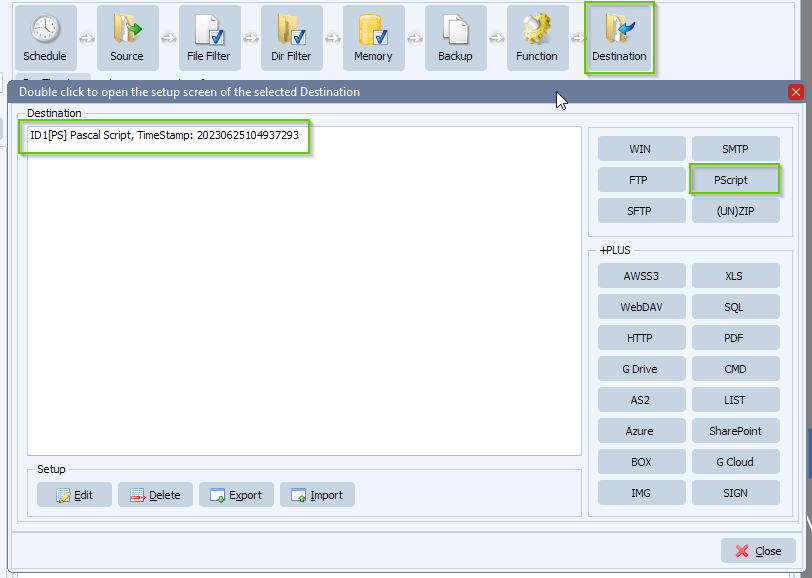
Begin
psExitCode:= 1;
// ... add your code here
psVIA := psVIA + 1;
End.
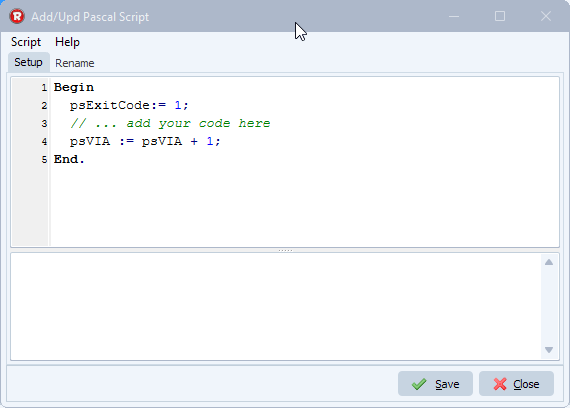
- Please open our “Rule Events” option:
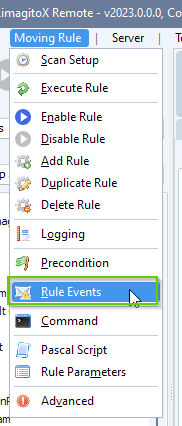
- Select and enable “On Rule Begin” event and enable “Enable Pascal Script”. Add the following script which will reset our internal counter psVIA when the Rule begins scanning.
Begin
psExitCode:= 1;
// ... add your code here
psVIA := 0;
End.
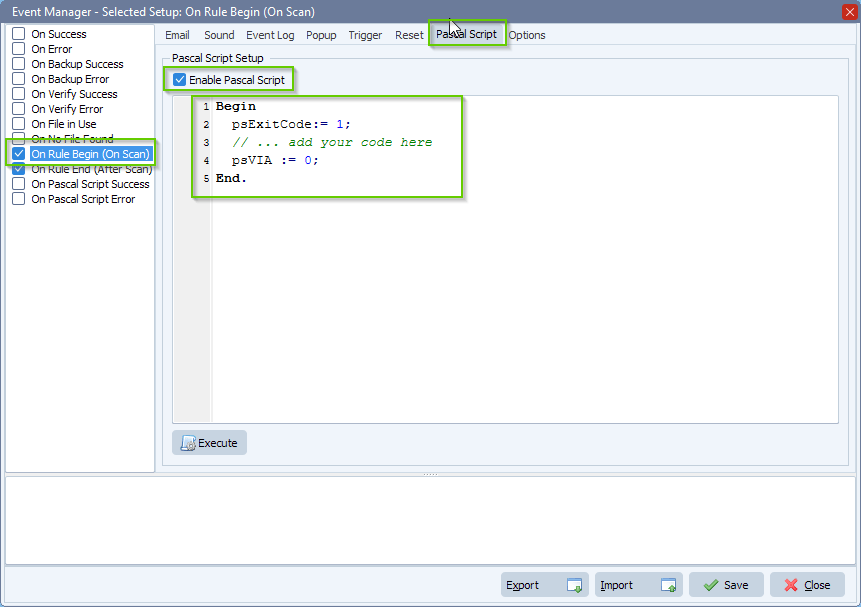
- Select and enable “On Rule End” event and enable “Enable Pascal Script”. Add the following script. We use a second internal variable psVIB to create a memory function regarding the sending of the email.
Const
ctMaxFilesAllowed = 5;
Begin
psExitCode:= 0;
// ... add your code here
If (psVIA > ctMaxFilesAllowed) Then
Begin
If psVIB = 0 Then
Begin
psVIB := 1;
psExitCode := 1;
psLogWrite(1, '', 'Send Email, Max files reached');
End;
End
Else
psVIB := 0;
End.
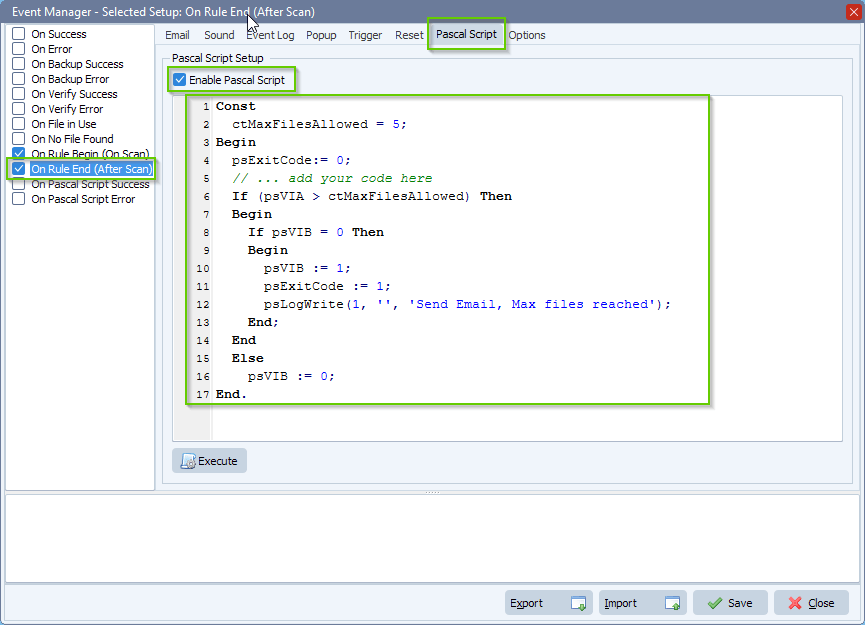
- Be sure to enable the “Use Pascal Script as Event Precondition” under Options for the “On Rule End’ event. The result of the Pascal Script (psExitCode) will determine of an email will be sent or not.
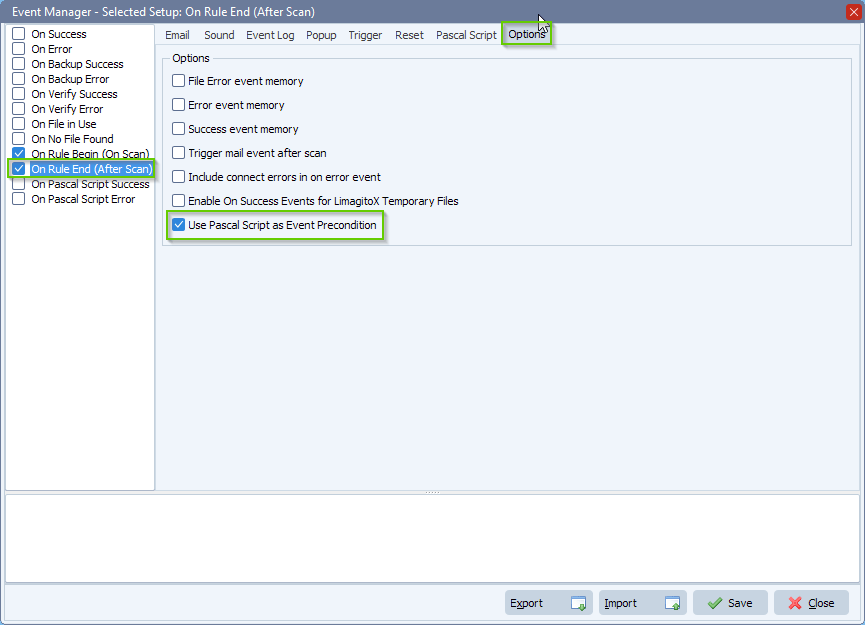
- Setup email for the “On Rule End” event.
- Enable “Enable Mail” option
- Setup Email
- Setup Common (or Rule) SMTP. The Common setup can be used by other Rules and is mostly used.
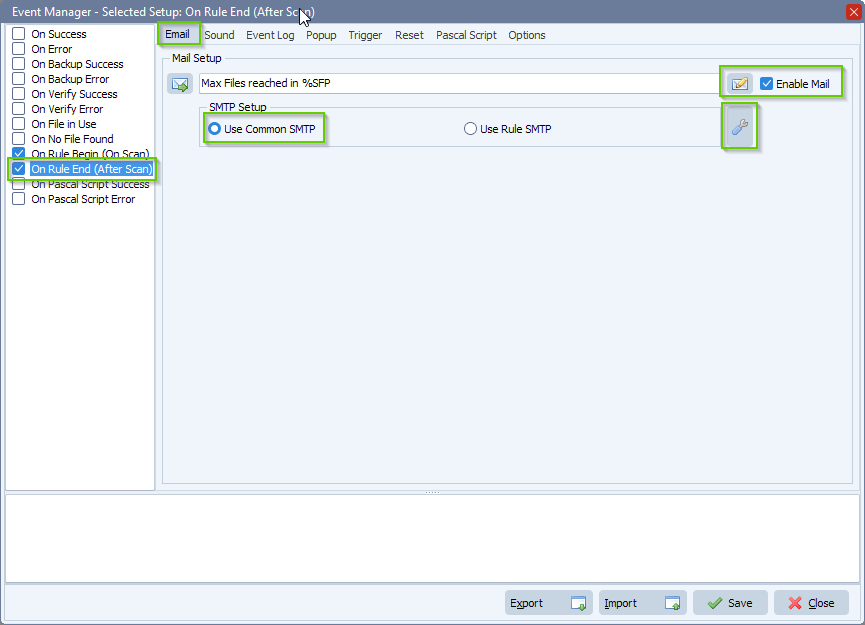
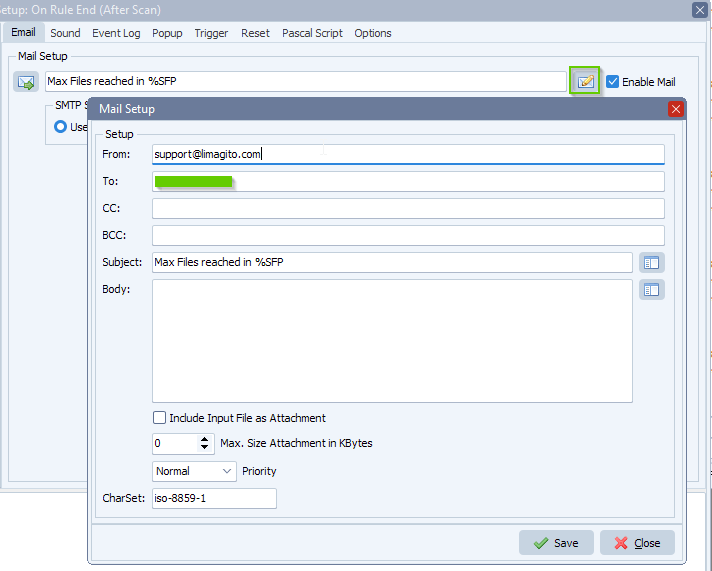
- We are using the Google SMTP server in this example:
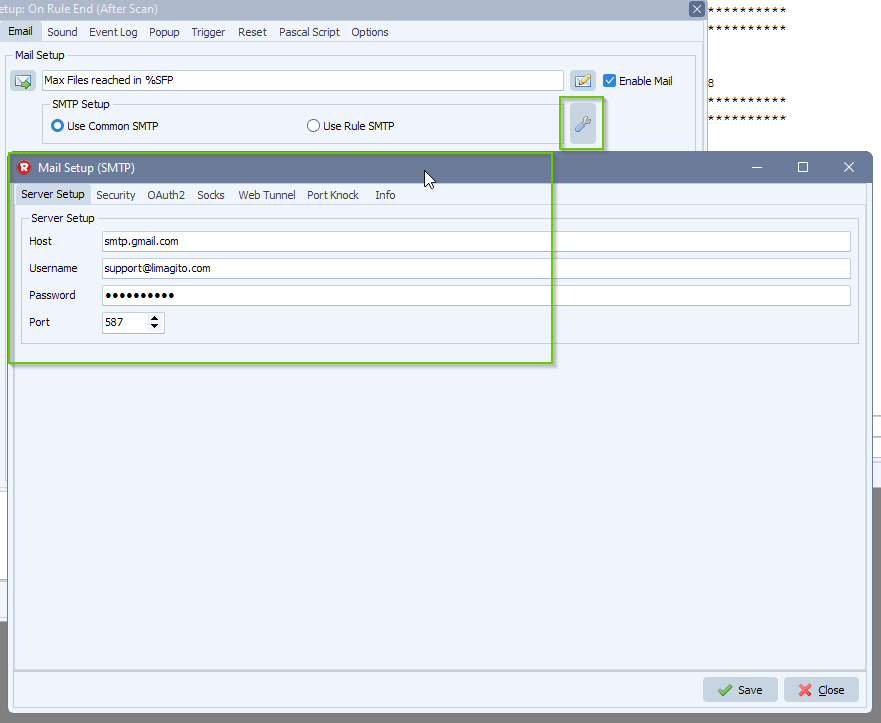
- RunTime Log result:
- The first time the Rule was triggered, it found more than 5 files and an email was sent.
- The second time the amount of files was still more than 5 files but we didn’t sent an email. We will wait until the amount of files is lower again to reset this memory status.
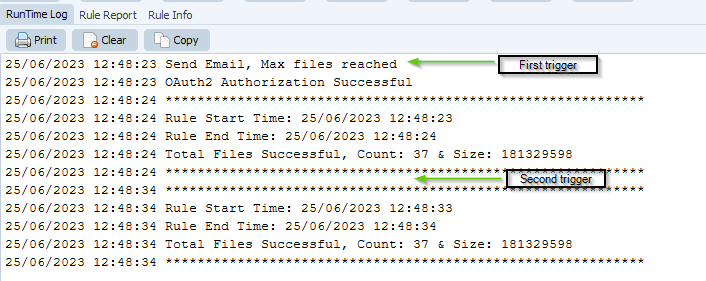
#Filetransfer #SMTP
If you need any info about this “alert me if files haven’t moved” option, please let us know.
Best regards,
Limagito Team